This is a tutorial designed to help new game developers create a simple slot machine game using Lanica’s Platino Game Engine. The end result will look something like this, and the process will teach you about creating a scene in Platino, spritesheets, basic Javascript, variables, touch events, displaying and updating text, and dragging objects.
rolls[random -1]++;
This will *greatly* decrease the verbosity of your program, and make it more clear why it's doing what it does.
Stephan van Hulst wrote:First of all, start by making your program more clear by eliminating all the 'cases' you use. You can store your values in a simple array like this:
rolls[random -1]++;
This will *greatly* decrease the verbosity of your program, and make it more clear why it's doing what it does.
I can't use rolls to store my values, rolls needs to be a number put in by the user because it determines how many times a dice would be rolled. The only real use I have for it is to determine when the main for loop ends. Then I need compare each new random to 1-12, to determine how many times that number has been rolled. I sort of understand what you're getting at with rolls[random -1]++ but I'm not quite sure how to assign the array as you're trying to point out.
Anyways this is what I've worked out according to what I think you mean. Still get the same issue though. I think it's asking for a new rolls every time the for loop runs through, any idea how to fix that?
Anyway, looking at your program, I don't see why it would keep asking for input. You should have an entirely different problem on your hand, namely that you're stuck in a permanent loop. Have a look at the exit condition of your nested for loop.
By the way, you can also eliminate a lot in your display code.
If I may give you a big hint, I think you are using the nested loop for the wrong purpose. Tell me, how many dice do you have?
Stephan van Hulst wrote:I apologize, I didn't notice you already had a variable with that name. I meant a new variable, which you have interpreted correctly.
Anyway, looking at your program, I don't see why it would keep asking for input. You should have an entirely different problem on your hand, namely that you're stuck in a permanent loop. Have a look at the exit condition of your nested for loop.
By the way, you can also eliminate a lot in your display code.
If I may give you a big hint, I think you are using the nested loop for the wrong purpose. Tell me, how many dice do you have?
That's the format the displays supposed to go in, according to the example given by my teacher. These are the assignment instructions, and I have to use nested loops because of the section I'm on, it's an Ap class that i take online. So it's mostly self learned.
1. Create a new project called 5.05 Random Dice in the Mod05
Assignments folder.
2. Create a class called DiceProbability in the newly created project
folder.
3. Ask the user to input how many times the dice will be rolled.
4. Calculate the probability of each combination of dice. (You may want to start with
more familiar six-sided dice.)
5. Print the results neatly in two columns (do not worry about excessive decimal places).
6. What is the effect on the percentages when the number of rolls is increased?
7. After the program works, you might want to make it more interesting and ask the user
to enter the number of sides on a die (singular for dice).
You can make your display code display the same stuff, except with less verbose code, in the same way you altered the rest of your program. 'Two or more, use a for'.
Stephan van Hulst wrote:It doesn't say in the requirements you need a nested loop.
It isn't very specific but it does say it in the grading rubric, and the assignment is going to be turned in as Assignment 5.05 nested loops. So I'm pretty sure it had to use nested loops, believe me if it was my choice I wouldn't use nested loops for this.

Javascript Slot Machine
1. Ask the number of rolls
2. Make a new array which is the size of the number of rolls. Each element will contain the roll result
3. Roll the dice and store the result in the array
4. Loop through each dice face (by the way, the numbers of faces should be kept in a variable)
5. Loop through the array, and count the number of times the current face was rolled
6. Calculate the probability the current faced has been rolled
It should do this:
This will calculate probabilities. Your program right now just rolls a d12 and this will, with enough rolls, come close to the probability, but will not show it.
Life is full of choices. Sometimes you make the good ones, and sometimes you have to kill all the witnesses.
I was bored and that can be a dangerous thing. Like doodling on the phone book while you are talking on the phone, I doodle code while answering questions on DIC. Yeah, it means I have no life and yes it means I was born a coder. During this little doodle I decided to make a slot machine. But not your standard slot machine per say, but one designed a little bit more like the real thing. Sure it could have been done a little more simpler and not even using a Wheel class at all, but what fun is that? In this entry I show the creation of a slot machine from a bit more of a mechanical aspect than a purely computerized one. It should provide a small sampling of classes and how they can represent real life machines. We cover it all right here on the Programming Underground!
So as I have already said, this little project was just something to play around with. It turned out kinda nice, so I thought I would share it. But what did I mean about it being mechanical in nature? Well, if you have ever played a real slot machine, not the digital ones they have in casinos now, you would see a metal case with a series of wheels. Typically it would be three wheels with pictures on them. When you put your money in and pull the handle the wheels would be set into motion. They would spin and then the first wheel would stop, followed by the second and then the third. After they have all stopped, the winnings are determined and you are paid out in coinage or credits.
I thought, why not be a bit mechanical in this slot machine design and create the wheels as a class called “Wheel” and give it the ability to spin independently of the other wheels? Have the wheel keep track of which picture (or in our case number) is flying by and report the results to the actual slot machine class. I could have done this mechanism without the need of a wheel at all and instead load up an array and have it randomly pick a number from the wheel. Little slimmer, little more efficient but wouldn’t show much programming theory.
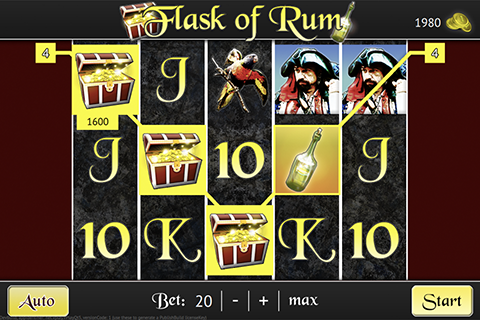
What do we gain by recreating these Wheel classes and spinning them independently? Well, you gain a slight bit of flexibility. Independently we are able to control the speed of the spinning if we wanted to, we are able to grasp the idea of the wheel as a concept in our mind and manipulate it. We could easily built in features like if the wheel lands on a certain number it will adjust itself. Like some slots in Vegas, if you land on lets say a rocket in the center line, the machine would see the rocket and correct the wheel to spin backwards 1 spot (in the direction of the rocket as if the rocket was controlling the wheel). We could spin one wheel one way and another wheel another. We could inherit from that wheel and create a specialized wheel that does a slew of new different behaviors. All encapsulated into one solid object making the actual Machine class oblivious to the trickery of the wheel itself… encapsulation at its finest!
The machine class we create will contain 3 pointers. Each to one of the wheels. The machine itself will be in charge of a few different tasks. Taking money, issuing and removing credits, determining when to spin, telling each of the wheels to spin and checking our winnings based on some chart we create. It has enough on its plate than worrying about the wheels and reading their values.
So lets start with our Wheel class and its declaration/implementation…
wheel.h
As you can see the wheel itself is not a difficult concept to envision. The bulk of the work is in the read() method. Here we simply read the values from our internal array of integers (the values on the wheel) and return those values as an array of the three integers… representing the visible column. This column will then be loaded into our 2-Dimensional Array back in the Machine class. The 2D array represents the view or screen by which the user sees the results. Remember that the user never gets to see the entire wheel. Only the 3 consecutive values on the face of the wheel.
Here is how it may look in the real world. We have our machine with the three wheels and our 2D array called “Screen” which acts as our viewing window. Each wheel will report its values and those values will be put into the screen…
Below is our machine class…
machine.h
This looks like a lot of code but really it is not if you look at each function. Most of them are very very simple to understand. We have a spin method which essentially spins each of the wheels, reads their values back from the Wheel class into a pointer (representing each column), then they are loaded into the 2D array one column at a time (our view screen), printed for the user to see the results and lastly the winnings are checked. The checkwinnings() method determines which rows to check based on the amount of the bet. If they chose 1 line, it checks for winning combinations on the middle row only. If they choose 2 lines, it checks the middle and top lines, 3 line bet checks all three horizontal rows, 4 line bet checks the first diagonal as well and 5 line bet checks both diagonals in addition to the lines.
How does it check the lines? Well each line is given to the checkline() helper function which compares the 3 values of the line against an enumerated type of various symbols. Here we are just assigning a symbol against each numbered value to help the programmer determine which numbers correspond to which winning combos. For instance, luckyseven represents the number 3 in the enumeration. So if it runs across a line with 3 number 3s, then it knows it hit the grand jackpot and credits the player 1000. This method makes things easy because if we ever wanted to change the win patterns later, we could change the enum and checkline method to do so. We could also build in multiple types of symbols and even let the user choose what slot machine game they want to go by. It becomes very flexible and is a testament to great design!
Lastly we can put some tests together just to show some the various aspects of how this thing works and how the programmer can use the classes…
slotmachine.cpp
Java Program Slot Machine Example Software
This simply inserts a 5 dollar bill and a coin for good luck. Then bets 5 lines and spins. Despite the outcome we go and bet five lines again and spin once more. Hopefully we win something this time around! But either way, those are the classes for you and I hope you like them. As always, all code here on the Programming Underground is in the public domain and free for the taking (just don’t cause a mess in isle 3, I am tired of running out there for cleanup). Thanks for stopping by and reading my blog. 🙂